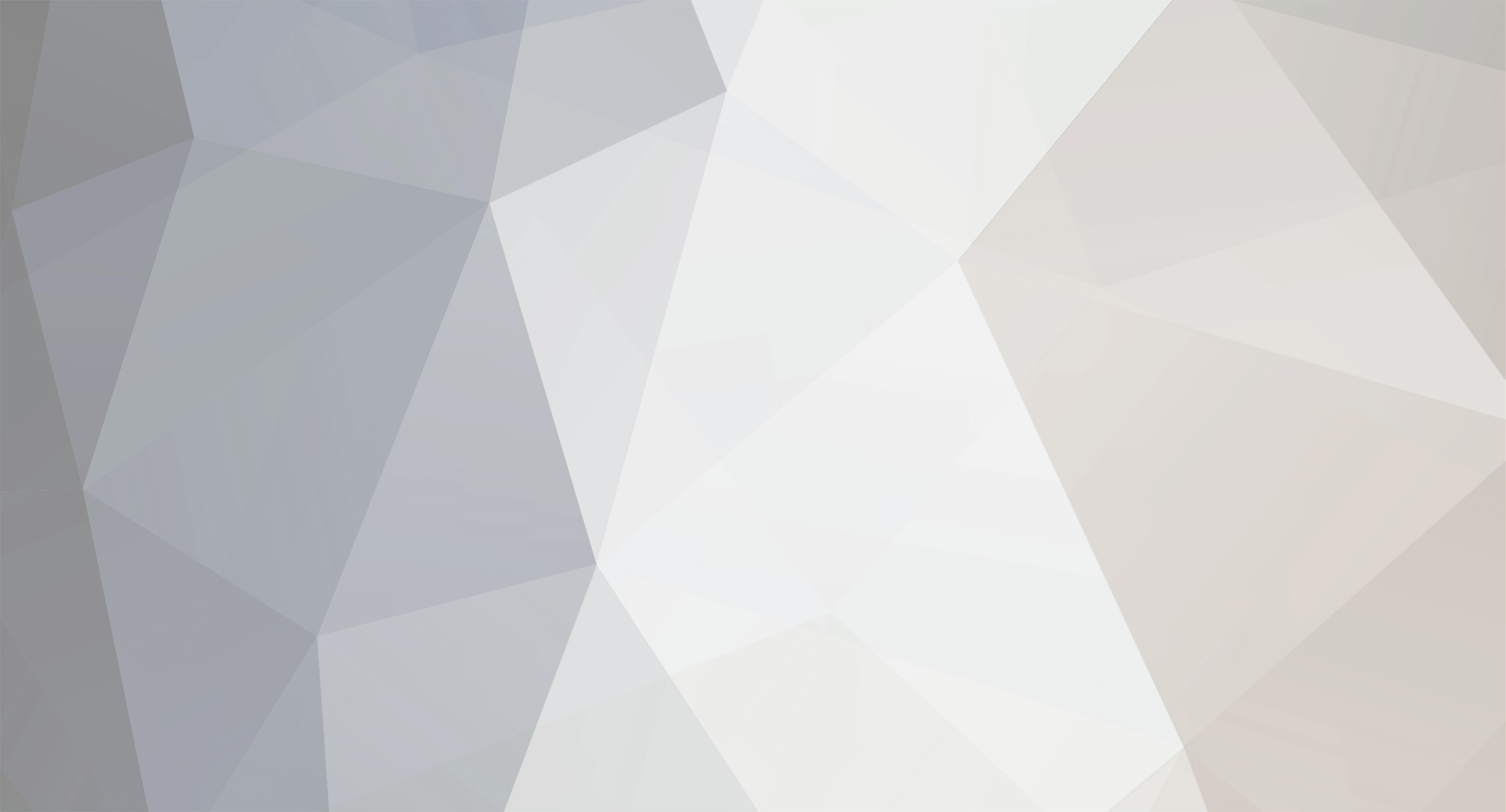
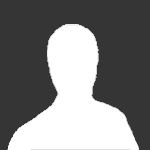
gen1us2k
-
Posts
20 -
Joined
-
Last visited
Never -
Donations
0.00 GBP
Content Type
Bug Tracker
Wiki
Release Notes
Forums
Downloads
Blogs
Events
Posts posted by gen1us2k
-
-
so. I need to create a character and set char's data in config file or not?
PS. lookup code and maybe I'll understand.
sorry for my english
-
add code from *.rej file...
sorry, but I patching sources, and add from rejects with my hands
PS maybe later i post the correct patch
-
for MaNGOS 9565. not tested yet
diff --git a/src/game/Map.cpp b/src/game/Map.cpp index befc8e1..2ac75c4 100644 --- a/src/game/Map.cpp +++ b/src/game/Map.cpp @@ -926,11 +926,36 @@ Map::CreatureRelocation(Creature *creature, float x, float y, float z, float ang creature->SetNeedNotify(); } } + // hack for eye of acherus part 1 + if(creature->isCharmed()) + { + NGridType* oldGrid = getNGrid(old_cell.GridX(), old_cell.GridY()); + RemoveFromGrid(creature->GetCharmerOrOwnerPlayerOrPlayerItself(), oldGrid, old_cell); + if(!old_cell.DiffGrid(new_cell)) + AddToGrid(creature->GetCharmerOrOwnerPlayerOrPlayerItself(), oldGrid, new_cell); + else + EnsureGridLoadedAtEnter(new_cell, creature->GetCharmerOrOwnerPlayerOrPlayerItself()); + } } else { creature->Relocate(x, y, z, ang); creature->SetNeedNotify(); + // hack for eye of acherus part 2 + if(creature->isCharmed()) + { + UpdatePlayerVisibility(creature->GetCharmerOrOwnerPlayerOrPlayerItself(), new_cell, new_val); + UpdateObjectsVisibilityFor(creature->GetCharmerOrOwnerPlayerOrPlayerItself(), new_cell, new_val); + PlayerRelocationNotify(creature->GetCharmerOrOwnerPlayerOrPlayerItself(), new_cell, new_val); + + bool same_cell = (new_cell == old_cell); + NGridType* newGrid = getNGrid(new_cell.GridX(), new_cell.GridY()); + if( !same_cell && newGrid->GetGridState()!= GRID_STATE_ACTIVE ) + { + ResetGridExpiry(*newGrid, 0.1f); + newGrid->SetGridState(GRID_STATE_ACTIVE); + } + } } assert(CheckGridIntegrity(creature,true)); diff --git a/src/game/Object.cpp b/src/game/Object.cpp index 9ec6a5b..9de6ded 100644 --- a/src/game/Object.cpp +++ b/src/game/Object.cpp @@ -1453,6 +1453,10 @@ void WorldObject::MonsterTextEmote(const char* text, uint64 TargetGuid, bool IsB { WorldPacket data(SMSG_MESSAGECHAT, 200); BuildMonsterChat(&data,IsBossEmote ? CHAT_MSG_RAID_BOSS_EMOTE : CHAT_MSG_MONSTER_EMOTE,text,LANG_UNIVERSAL,GetName(),TargetGuid); + Unit * eye = Unit::GetUnit((*this), TargetGuid); + if (eye && eye->isCharmed() && eye->GetEntry() == 28511) + SendMessageToSet(&data, true); + else SendMessageToSetInRange(&data,sWorld.getConfig(IsBossEmote ? CONFIG_FLOAT_LISTEN_RANGE_YELL : CONFIG_FLOAT_LISTEN_RANGE_TEXTEMOTE),true); } diff --git a/src/game/Spell.h b/src/game/Spell.h index a09c7c0..1c9a2e0 100644 --- a/src/game/Spell.h +++ b/src/game/Spell.h @@ -241,6 +241,7 @@ class Spell friend void Unit::SetCurrentCastedSpell( Spell * pSpell ); public: + void EffectSummonPosessed(SpellEffectIndex eff_idx); void EffectEmpty(SpellEffectIndex eff_idx); void EffectNULL(SpellEffectIndex eff_idx); void EffectUnused(SpellEffectIndex eff_idx); diff --git a/src/game/SpellEffects.cpp b/src/game/SpellEffects.cpp index 9c7d8f8..b303eb1 100644 --- a/src/game/SpellEffects.cpp +++ b/src/game/SpellEffects.cpp @@ -3788,9 +3788,20 @@ void Spell::EffectSummonType(SpellEffectIndex eff_idx) } case SUMMON_PROP_GROUP_CONTROLLABLE: { + switch(prop_id) + { + //SUMMON_TYPE_POSESSED = 65 + //SUMMON_TYPE_POSESSED2 = 428 + case 65: + case 428: + EffectSummonPosessed(eff_idx); + break; + default: DoSummonGuardian(eff_idx, summon_prop->FactionId); + break; + } // no type here // maybe wrong - but thats the handler currently used for those - DoSummonGuardian(eff_idx, summon_prop->FactionId); + // DoSummonGuardian(eff_idx, summon_prop->FactionId); break; } case SUMMON_PROP_GROUP_VEHICLE: @@ -3805,6 +3816,61 @@ void Spell::EffectSummonType(SpellEffectIndex eff_idx) } } +void Spell::EffectSummonPosessed(SpellEffectIndex eff_idx) +{ + uint32 creature_entry = m_spellInfo->EffectMiscValue[eff_idx]; + if (!creature_entry) + return; + + int32 duration = GetSpellDuration(m_spellInfo); + + float px, py, pz; + // If dest location if present + if (m_targets.m_targetMask & TARGET_FLAG_DEST_LOCATION) + { + // Summon 1 unit in dest location + px = m_targets.m_destX; + py = m_targets.m_destY; + pz = m_targets.m_destZ; + } + // Summon if dest location not present near caster + else + m_caster->GetClosePoint(px, py, pz, 1.0f); + + TempSummonType summonType = (duration == 0) ? TEMPSUMMON_DEAD_DESPAWN : TEMPSUMMON_TIMED_OR_DEAD_DESPAWN; + Creature *spawnCreature = m_caster->SummonCreature(creature_entry, px, py, pz, m_caster->GetOrientation(), summonType, duration); + + if(!spawnCreature->IsPositionValid()) + { + sLog.outError("Pet (guidlow %d, entry %d) not created base at creature. Suggested coordinates isn't valid (X: %f Y: %f)", + spawnCreature->GetGUIDLow(), spawnCreature->GetEntry(), spawnCreature->GetPositionX(), spawnCreature->GetPositionY()); + delete spawnCreature; + return; + } + + spawnCreature->setFaction(m_caster->getFaction()); + spawnCreature->SetCharmerGUID(m_caster->GetGUID()); + spawnCreature->SetCreatorGUID(m_caster->GetGUID()); + + CharmInfo *charmInfo = spawnCreature->InitCharmInfo(spawnCreature); + charmInfo->InitPossessCreateSpells(); + + if(m_caster->GetTypeId()==TYPEID_PLAYER) + { + ((Player*)m_caster)->SetCharm(spawnCreature); + ((Player*)m_caster)->SetFarSightGUID(spawnCreature->GetGUID()); + ((Player*)m_caster)->SetClientControl(spawnCreature, 1); + ((Player*)m_caster)->SetMover(spawnCreature); + ((Player*)m_caster)->PossessSpellInitialize(); + } + + spawnCreature->SetFlag(UNIT_FIELD_FLAGS, UNIT_FLAG_PLAYER_CONTROLLED); + spawnCreature->SetFlag(UNIT_FIELD_FLAGS, UNIT_FLAG_NON_ATTACKABLE); + + spawnCreature->CombatStop(); + spawnCreature->DeleteThreatList(); +} + void Spell::DoSummon(SpellEffectIndex eff_idx) { if (m_caster->GetPetGUID()) @@ -5308,6 +5374,45 @@ void Spell::EffectScriptEffect(SpellEffectIndex eff_idx) { switch(m_spellInfo->Id) { + case 52694: // Recall Eye of Acherus + { + if(!m_caster || m_caster->GetTypeId() != TYPEID_UNIT || !(m_caster->isCharmed())) + return; + + Creature *eye = ((Creature*)m_caster); + if(m_caster->GetCharmer()->GetTypeId() != TYPEID_PLAYER) + return; + + Player *player =((Player*)m_caster->GetCharmer()); + if(eye->isInCombat()) + return; + + eye->GetMap()->CreatureRelocation(eye, 2325.0f, -5660.0f, 427.0f, 3.83f); + eye->RemoveFlag(UNIT_FIELD_FLAGS, UNIT_FLAG_PLAYER_CONTROLLED); + eye->SetCharmerGUID(0); + + player->InterruptSpell(CURRENT_CHANNELED_SPELL); + player->RemoveAurasDueToSpell(51852); // Remove The Eye of Acherus aura + player->RemoveAurasDueToSpell(51923); + player->RemoveAurasDueToSpell(51890); + player->SetCharm(NULL); + player->SetFarSightGUID(0); + player->SetClientControl(m_caster, 0); + player->SetMover(NULL); + player->RemovePetActionBar(); + + eye->CleanupsBeforeDelete(); + eye->AddObjectToRemoveList(); + return; + } + case 51904: // Summon Ghouls On Scarlet Crusade + { + if(!unitTarget) + return; + + unitTarget->CastSpell(unitTarget, 54522, true); + break; + } case 8856: // Bending Shinbone { if (!itemTarget && m_caster->GetTypeId()!=TYPEID_PLAYER)
-
git clone git://github.com/jolan/MoveMaps.git
-
for MaNGOS_rev9471
-
Patch work??
need up-to-date
-
Check you configuration.php for correct values.
???
have you changed this file for you?
google for 500 internal server error
-
so .. sorry .. mangos now officially supports 3,2,2 and 3,3,0, or switched to?
Have not dealt with mangos and histori-project commits read broke:)
Shl thanks for added me:)
-
U can seat on tanks but its works nemnogo koryavo
in Ulduar vehicles operate so.
you can ride on them
you can shoot
but they have not filled with energy
-
here my vehicle data.... http://pastie.org/740244 Vehicles in Ulduar works normally (pa4ti)
be useful
-
ulduar's vehicle done... look for correctly work ulduar vehicles
-
So, I understood that basically vehicles in Ulduar are realized ... all vehicles are working normally ... It's only deal with spawn left.
PS now I'm picking base of 3x repositories ... repository Kicho, current repository and the base with an alternative kernel ...
PPS I don't want to put trinitycore2 because there already almost is done ... It's better to look at code
-
tnx... really,,, The Argent Tournament is works
i take data from http://github.com/kicho/vehicle/raw/master/sql/Vehicle/mangos_vehicle_sql.sql
and ulduar'd vehicle works... but.... O see them on;y why I'm in GM mode...
-
compiled...
allied updates from mangos & vehicle....
@ ulduar its dont work...
@ wintergrasp too
I think that vehicles dont work on me
petbat ok.... I'll see ur sql file.,,,
vehicles dont work.,,, maybe i not correct pacthed my database? O_o
-
but... I understood one thing.
I got a clean source... Then
git pull git://github.com/Tasssadar/Valhalla-Project.git vehicle
then
patch -p1 -l <dua.patch
now compiling... then testing...
and I notices 3-4 SQL errors,,,
but a made vehicle.pacth as diff master vehicle....
Well ... see how It Will work
sorry for my bad English
is difference as I apply updates from vehicles previous to MaNGOS updates or not?
-
Im recompiled 5 times... doesn't work... maybe i do anything wrong
,,, Ok I test now another way
-
Kind time of the days, Respected.
I will ask the help to instal to me this patch on "8932" version of acore. There are errors at flood filling. I ask a pardon for my English. Has read many posts and messages, but has not understood. In advance thanks! To me it is very important.
git clone git://github.com/mangos/mangos.git cd mangos git checkout -b vehicle git pull git://github.com/Tasssadar/Valhalla-Project.git vehicle git diff -p master vehicle > vehicle.patch cp vehicle.patch ../path/to/your/patches git checkout master patch -p1 -l < ../path/to/your/patches/dualspec.patch patch -p1 -l < ../path/to/your/patches/vehicle.patch
I do so...
-
MaNGOS rev 8913..
U dont need patches... ITs work perfectly...
Clean sources
-
NetSky
Maybe you give link to your git repo?
[Patch] Auction House Bot (Xeross' Branch)
in OldCore modifications
Posted
ok. thanks for your answers. I will try make AHBot work today.