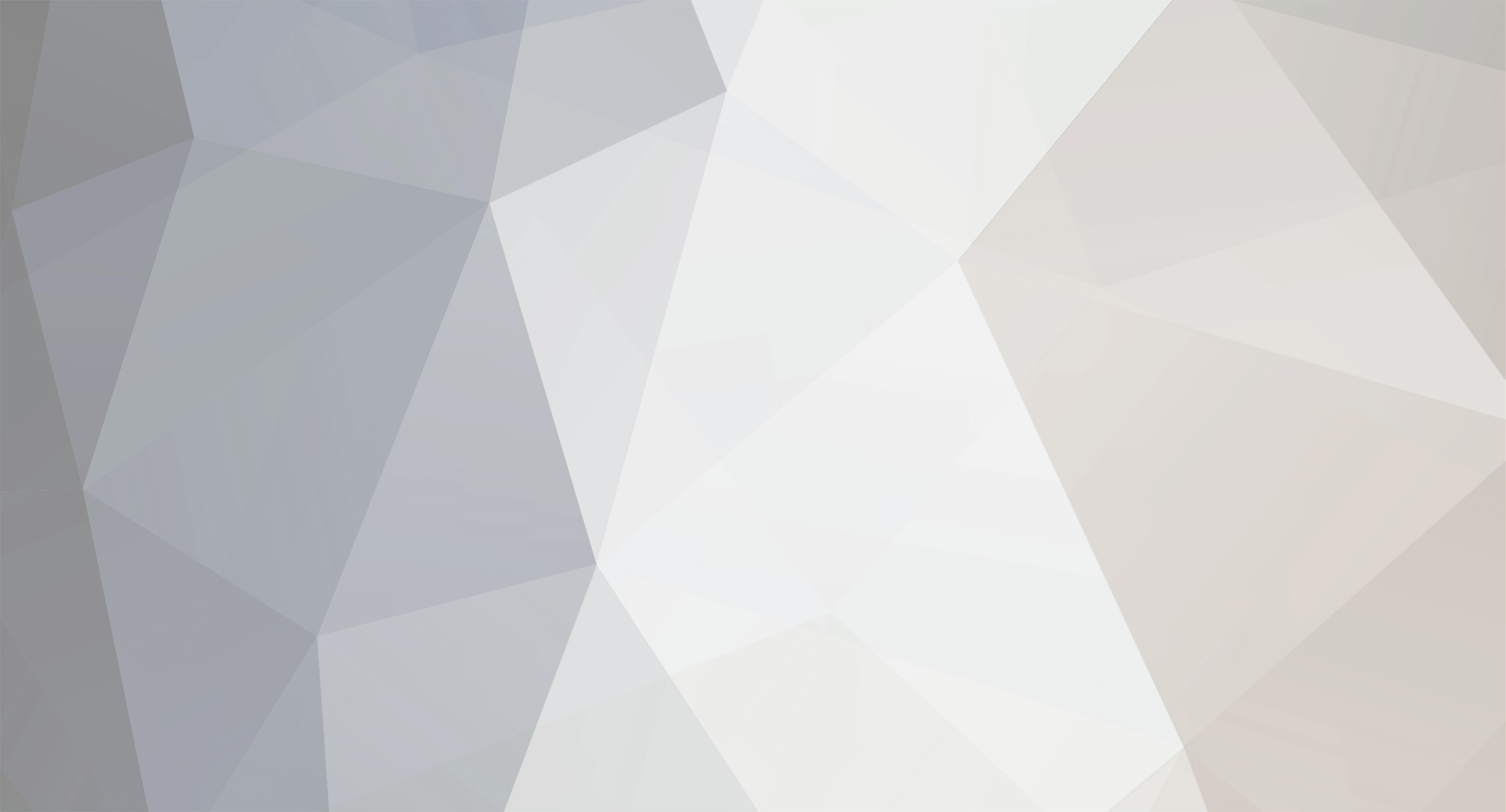
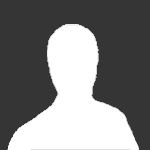
Kroket
-
Posts
52 -
Joined
-
Last visited
Never -
Donations
0.00 GBP
Content Type
Bug Tracker
Wiki
Release Notes
Forums
Downloads
Blogs
Events
Posts posted by Kroket
-
-
Im creating a donator shop via Gossip and would like to display text such as item information, price etc as normal text and not a menu item.
How would i do that?
-
Looks very nice, i have a site just like this but ofcourse mine is no framework but it also got a Template Engine.
Does yours also have a Database & Site Engine?
-
noshit, but how with scriptdev2?
-
Im creating a donator shop via Gossip and would like to display text such as item information, price etc as normal text and not a menu item.
How would i do that?
-
lmfao
-
Are you sure? few years ago when i had an arcemu server i managed to add it infront of the player name.
There also was this server that added people's country code infront of their name (if im not mistaken).
-
AH ok, i thought i would attempt to make a global world channel.
-
if(ChannelMgr* cMgr = channelMgr(GetTeam())) { if(Channel *chn = cMgr->GetJoinChannel("GLOBAL", 0)) { chn->Join(GetGUID(), NULL); chn->SetAnnounce(true); chn->Announce(GetGUID()); chn->Say(GetGUID(), "Hi everyone!", 0); } }
The player joins the channel but:
#1 it doesnt get announced.
#2 cannot send any messages in that channel.
-
if(type == CHAT_MSG_SAY) { if(GetPlayer()->isDonator()) msg.insert(0, "[Donator] "); GetPlayer()->Say(msg, lang); }
But now it appears INFRONT of the message and not infront of the player name.
-
void Player::Say(const std::string& text, const uint32 language) { WorldPacket data(SMSG_MESSAGECHAT, 100); if(isDonator()) text.insert(0, "[Donator] "); BuildPlayerChat(&data, CHAT_MSG_SAY, text, language); SendMessageToSetInRange(&data,sWorld.getConfig(CONFIG_FLOAT_LISTEN_RANGE_SAY),true); }
-
Sure, you can close this btw
-
..\\..\\src\\game\\Player.cpp(15796) : error C2663: 'std::basic_string<_Elem,_Traits,_Ax>::insert' : 10 overloads have no legal conversion for 'this' pointer
2> with
2> [
2> _Elem=char,
2> _Traits=std::char_traits<char>,
2> _Ax=std::allocator<char>
2> ]
-
Cool! i will try it.
-
I tried:
void Player::Say(const std::string& text, const uint32 language) { WorldPacket data(SMSG_MESSAGECHAT, 100); if(isDonator()) { std::string prefix = "[Donator] "; data << prefix.length()+1; data << prefix; } BuildPlayerChat(&data, CHAT_MSG_SAY, text, language); SendMessageToSetInRange(&data,sWorld.getConfig(CONFIG_FLOAT_LISTEN_RANGE_SAY),true); }
But that wont work.
-
How would i add an prefix to players that meet certain requirements such as being a donator.
Example: [Donator] pName Says: Hi!
I can just add the prefix to the world packet?
-
If i do that it asks me to enter details? i type something in and press enter and nothing happens.
-
Didnt bother to search lol.
Anyways resolved:
git stash git pull git stash apply
-
I know about git pull but it wont update Player.cpp cause i modified it.
How can i merge it and not lose any changes?
-
This forum is more active so here is the chance to get a answer bigger.
-
MangosZero
ScriptDevZero
http://www.wowhead.com/npc=14509#comments what is described here should be scripted for Thekal but apparantly its not.
If one of the 3 dies then they should be ressurected but that doesn't work (using .die).
-
CalculateShaPassHash calculates the wrong sha1 hash and this causes the authentication failure.
I found this out by printing the username, accid, password and sha1 hashed password in the console.
All matched except the hashed password.
-
this piece of code handles authentication:
///[*] If the input is '<password>' (and the user already gave his username) case LG: { //login+pass ok std::string pw = inputBuffer; if (sAccountMgr.CheckPassword(accId, pw)) { stage=OK; sendf("+Logged in.\\r\\n"); sLog.outRALog("User account %u has logged in.", accId); sendf("mangos>"); } else { ///- Else deny access sendf("-Wrong pass.\\r\\n"); sLog.outRALog("User account %u has failed to log in.", accId); if(bSecure) { handle_output(); return -1; } sendf("\\r\\n"); sendf(sObjectMgr.GetMangosStringForDBCLocale(LANG_RA_PASS)); } break;
-
Checked that already.
RASocket.cpp (MangosZero latest rev):
/* * Copyright (C) 2005-2010 MaNGOS <http://getmangos.eu/> * Copyright (C) 2009-2010 MaNGOSZero <http://github.com/mangoszero/mangoszero/> * * This program is free software; you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation; either version 2 of the License, or * (at your option) any later version. * * This program is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * GNU General Public License for more details. * * You should have received a copy of the GNU General Public License * along with this program; if not, write to the Free Software * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA */ /** \\file \\ingroup mangosd */ #include "Common.h" #include "Database/DatabaseEnv.h" #include "Log.h" #include "RASocket.h" #include "World.h" #include "Config/Config.h" #include "Util.h" #include "AccountMgr.h" #include "Language.h" #include "ObjectMgr.h" /// RASocket constructor RASocket::RASocket() :RAHandler(), pendingCommands(0, USYNC_THREAD, "pendingCommands"), outActive(false), inputBufferLen(0), outputBufferLen(0), stage(NONE) { ///- Get the config parameters bSecure = sConfig.GetBoolDefault( "RA.Secure", true ); bStricted = sConfig.GetBoolDefault( "RA.Stricted", false ); iMinLevel = AccountTypes(sConfig.GetIntDefault( "RA.MinLevel", SEC_ADMINISTRATOR )); reference_counting_policy ().value (ACE_Event_Handler::Reference_Counting_Policy::ENABLED); } /// RASocket destructor RASocket::~RASocket() { peer().close(); sLog.outRALog("Connection was closed."); } /// Accept an incoming connection int RASocket::open(void* ) { if (reactor ()->register_handler(this, ACE_Event_Handler::READ_MASK | ACE_Event_Handler::WRITE_MASK) == -1) { sLog.outError ("RASocket::open: unable to register client handler errno = %s", ACE_OS::strerror (errno)); return -1; } ACE_INET_Addr remote_addr; if (peer ().get_remote_addr (remote_addr) == -1) { sLog.outError ("RASocket::open: peer ().get_remote_addr errno = %s", ACE_OS::strerror (errno)); return -1; } sLog.outRALog("Incoming connection from %s.",remote_addr.get_host_addr()); ///- print Motd sendf(sWorld.GetMotd()); sendf("\\r\\n"); sendf(sObjectMgr.GetMangosStringForDBCLocale(LANG_RA_USER)); return 0; } int RASocket::close(int) { if(closing_) return -1; DEBUG_LOG("RASocket::close"); shutdown(); closing_ = true; remove_reference(); return 0; } int RASocket::handle_close (ACE_HANDLE h, ACE_Reactor_Mask) { if(closing_) return -1; DEBUG_LOG("RASocket::handle_close"); ACE_GUARD_RETURN (ACE_Thread_Mutex, Guard, outBufferLock, -1); closing_ = true; if (h == ACE_INVALID_HANDLE) peer ().close_writer (); remove_reference(); return 0; } int RASocket::handle_output (ACE_HANDLE) { ACE_GUARD_RETURN (ACE_Thread_Mutex, Guard, outBufferLock, -1); if(closing_) return -1; if (!outputBufferLen) { if(reactor()->cancel_wakeup(this, ACE_Event_Handler::WRITE_MASK) == -1) { sLog.outError ("RASocket::handle_output: error while cancel_wakeup"); return -1; } outActive = false; return 0; } #ifdef MSG_NOSIGNAL ssize_t n = peer ().send (outputBuffer, outputBufferLen, MSG_NOSIGNAL); #else ssize_t n = peer ().send (outputBuffer, outputBufferLen); #endif // MSG_NOSIGNAL if(n<=0) return -1; ACE_OS::memmove(outputBuffer, outputBuffer+n, outputBufferLen-n); outputBufferLen -= n; return 0; } /// Read data from the network int RASocket::handle_input(ACE_HANDLE) { DEBUG_LOG("RASocket::handle_input"); if(closing_) { sLog.outError("Called RASocket::handle_input with closing_ = true"); return -1; } size_t readBytes = peer().recv(inputBuffer+inputBufferLen, RA_BUFF_SIZE-inputBufferLen-1); if(readBytes <= 0) { DEBUG_LOG("read %u bytes in RASocket::handle_input", readBytes); return -1; } ///- Discard data after line break or line feed bool gotenter=false; for(; readBytes > 0 ; --readBytes) { char c = inputBuffer[inputBufferLen]; if (c=='\\r'|| c=='\\n') { gotenter=true; break; } ++inputBufferLen; } if (gotenter) { inputBuffer[inputBufferLen]=0; inputBufferLen=0; switch(stage) { /// <ul> [*] If the input is '<username>' case NONE: { std::string szLogin=inputBuffer; accId = sAccountMgr.GetId(szLogin); ///- If the user is not found, deny access if(!accId) { sendf("-No such user.\\r\\n"); sLog.outRALog("User %s does not exist.",szLogin.c_str()); if(bSecure) { handle_output(); return -1; } sendf("\\r\\n"); sendf(sObjectMgr.GetMangosStringForDBCLocale(LANG_RA_USER)); break; } accAccessLevel = sAccountMgr.GetSecurity(accId); ///- if gmlevel is too low, deny access if (accAccessLevel < iMinLevel) { sendf("-Not enough privileges.\\r\\n"); sLog.outRALog("User %s has no privilege.",szLogin.c_str()); if(bSecure) { handle_output(); return -1; } sendf("\\r\\n"); sendf(sObjectMgr.GetMangosStringForDBCLocale(LANG_RA_USER)); break; } ///- allow by remotely connected admin use console level commands dependent from config setting if (accAccessLevel >= SEC_ADMINISTRATOR && !bStricted) accAccessLevel = SEC_CONSOLE; stage=LG; sendf(sObjectMgr.GetMangosStringForDBCLocale(LANG_RA_PASS)); break; } ///[*] If the input is '<password>' (and the user already gave his username) case LG: { //login+pass ok std::string pw = inputBuffer; if (sAccountMgr.CheckPassword(accId, pw)) { stage=OK; sendf("+Logged in.\\r\\n"); sLog.outRALog("User account %u has logged in.", accId); sendf("mangos>"); } else { ///- Else deny access sendf("-Wrong pass.\\r\\n"); sLog.outRALog("User account %u has failed to log in.", accId); if(bSecure) { handle_output(); return -1; } sendf("\\r\\n"); sendf(sObjectMgr.GetMangosStringForDBCLocale(LANG_RA_PASS)); } break; } ///[*] If user is logged, parse and execute the command case OK: if (strlen(inputBuffer)) { sLog.outRALog("Got '%s' cmd.",inputBuffer); if (strncmp(inputBuffer,"quit",4)==0) return -1; else { CliCommandHolder* cmd = new CliCommandHolder(accId, accAccessLevel, this, inputBuffer, &RASocket::zprint, &RASocket::commandFinished); sWorld.QueueCliCommand(cmd); pendingCommands.acquire(); } } else sendf("mangos>"); break; ///[/list] }; } // no enter yet? wait for next input... return 0; } /// Output function void RASocket::zprint(void* callbackArg, const char * szText ) { if( !szText ) return; ((RASocket*)callbackArg)->sendf(szText); } void RASocket::commandFinished(void* callbackArg, bool success) { RASocket* raSocket = (RASocket*)callbackArg; raSocket->sendf("mangos>"); raSocket->pendingCommands.release(); } int RASocket::sendf(const char* msg) { ACE_GUARD_RETURN (ACE_Thread_Mutex, Guard, outBufferLock, -1); if(closing_) return -1; int msgLen = strlen(msg); if(msgLen+outputBufferLen > RA_BUFF_SIZE) return -1; ACE_OS::memcpy(outputBuffer+outputBufferLen, msg, msgLen); outputBufferLen += msgLen; if(!outActive) { if (reactor ()->schedule_wakeup (this, ACE_Event_Handler::WRITE_MASK) == -1) { sLog.outError ("RASocket::sendf error while schedule_wakeup"); return -1; } outActive = true; } return 0; }
-
Those that violate the code of conduct get banned without a single warning (flaming etc).
Mangos Remote Admin
in OldGeneral support
Posted
bump