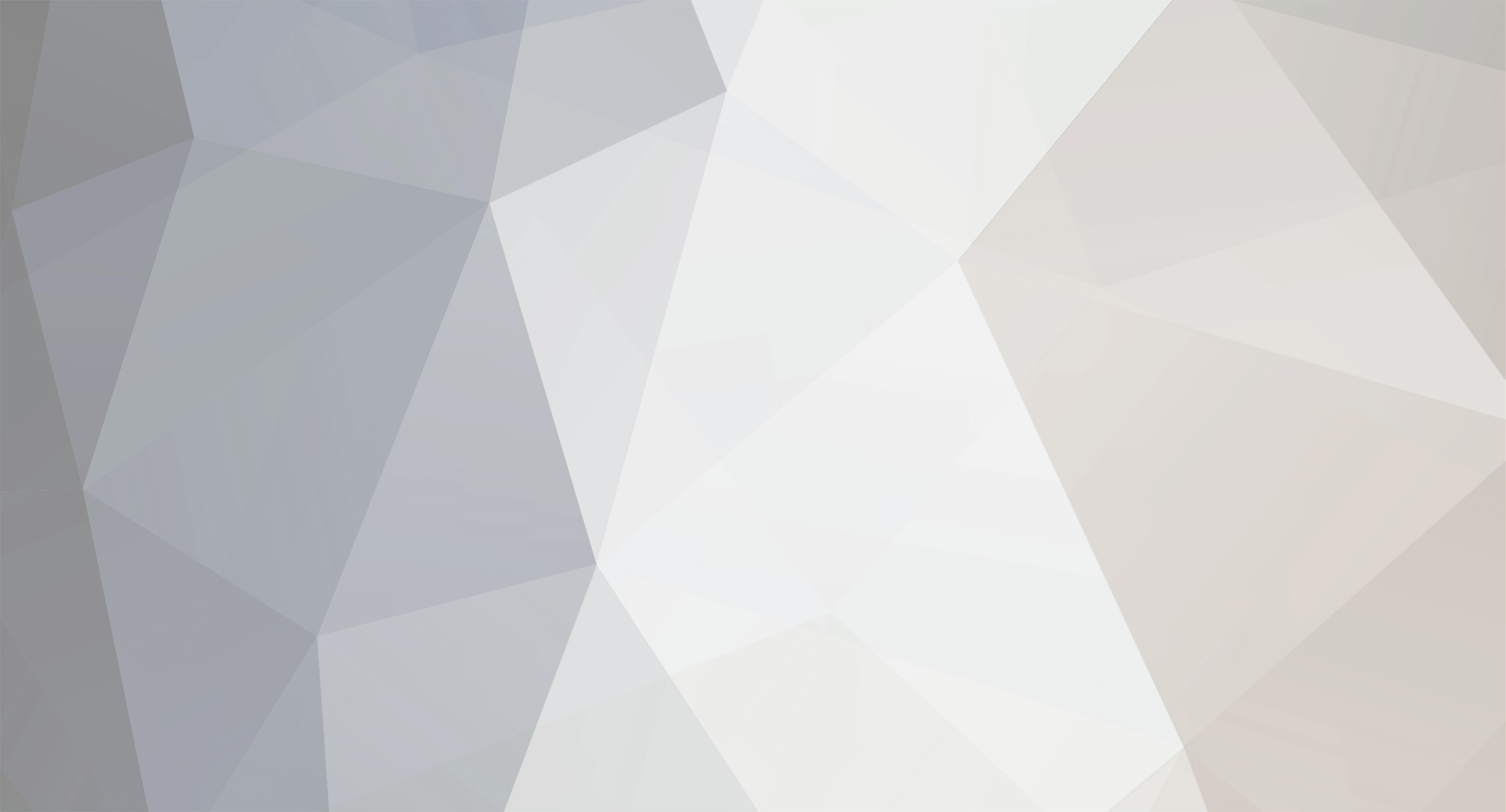
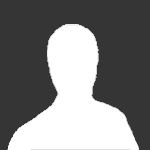
BThallid
Members-
Posts
63 -
Joined
-
Last visited
Never -
Donations
0.00 GBP
Content Type
Profiles
Bug Tracker
Wiki
Release Notes
Forums
Downloads
Blogs
Events
Everything posted by BThallid
-
I noticed that the HasPick function was checking database when the core already has compatible function. diff --git a/src/game/playerbot/PlayerbotAI.cpp b/src/game/playerbot/PlayerbotAI.cpp index 9fc8d4b..ab9e6de 100644 --- a/src/game/playerbot/PlayerbotAI.cpp +++ b/src/game/playerbot/PlayerbotAI.cpp @@ -2532,80 +2532,8 @@ bool PlayerbotAI::PickPocket(Unit* pTarget) bool PlayerbotAI::HasPick() { - QueryResult *result; - - // list out equiped items - for (uint8 slot = EQUIPMENT_SLOT_START; slot < EQUIPMENT_SLOT_END; slot++) - { - Item* const pItem = m_bot->GetItemByPos(INVENTORY_SLOT_BAG_0, slot); - if (pItem) - { - const ItemPrototype* const pItemProto = pItem->GetProto(); - if (!pItemProto) - continue; - - result = WorldDatabase.PQuery("SELECT TotemCategory FROM item_template WHERE entry = '%i'", pItemProto->ItemId); - if (result) - { - Field *fields = result->Fetch(); - uint32 tc = fields[0].GetUInt32(); - // sLog.outDebug("HasPick %u",tc); - if (tc == 165 || tc == 167) // pick = 165, hammer = 162 or hammer pick = 167 - return true; - } - } - } - - // list out items in backpack - for (uint8 slot = INVENTORY_SLOT_ITEM_START; slot < INVENTORY_SLOT_ITEM_END; slot++) - { - // sLog.outDebug("[%s's]backpack slot = %u",m_bot->GetName(),slot); // 23 to 38 = 16 - Item* const pItem = m_bot->GetItemByPos(INVENTORY_SLOT_BAG_0, slot); // 255, 23 to 38 - if (pItem) - { - const ItemPrototype* const pItemProto = pItem->GetProto(); - if (!pItemProto) - continue; - - result = WorldDatabase.PQuery("SELECT TotemCategory FROM item_template WHERE entry = '%i'", pItemProto->ItemId); - if (result) - { - Field *fields = result->Fetch(); - uint32 tc = fields[0].GetUInt32(); - // sLog.outDebug("HasPick %u",tc); - if (tc == 165 || tc == 167) // pick = 165, hammer = 162 or hammer pick = 167 - return true; - } - } - } - - // list out items in other removable backpacks - for (uint8 bag = INVENTORY_SLOT_BAG_START; bag < INVENTORY_SLOT_BAG_END; ++bag) // 20 to 23 = 4 - { - const Bag* const pBag = (Bag*) m_bot->GetItemByPos(INVENTORY_SLOT_BAG_0, bag); // 255, 20 to 23 - if (pBag) - for (uint8 slot = 0; slot < pBag->GetBagSize(); ++slot) - { - // sLog.outDebug("[%s's]bag[%u] slot = %u",m_bot->GetName(),bag,slot); // 1 to bagsize = ? - Item* const pItem = m_bot->GetItemByPos(bag, slot); // 20 to 23, 1 to bagsize - if (pItem) - { - const ItemPrototype* const pItemProto = pItem->GetProto(); - if (!pItemProto) - continue; - - result = WorldDatabase.PQuery("SELECT TotemCategory FROM item_template WHERE entry = '%i'", pItemProto->ItemId); - if (result) - { - Field *fields = result->Fetch(); - uint32 tc = fields[0].GetUInt32(); - // sLog.outDebug("HasPick %u",tc); - if (tc == 165 || tc == 167) - return true; - } - } - } - } + if (m_bot->HasItemTotemCategory(TC_MINING_PICK)) + return true; std::ostringstream out; out << "|cffffffffI do not have a pick!"; TellMaster(out.str().c_str()); The core's function also calls another function, IsTotemCategoryCompatiableWith, that checks if the item is compatible with the current TotemCategory, so it only has to be called for the specific category.
-
In server code, I do not see on either SMSG_RESURRECT_REQUEST or CMSG_RESURRECT_RESPONSE where it uses a packed guid. If a player releases, then no resurrection is possible. Or at least shouldn't be.
-
Thought I would add a bit more to the PriestAI and the resurrection thereof: diff --git a/src/game/playerbot/PlayerbotAI.cpp b/src/game/playerbot/PlayerbotAI.cpp index 53b1f44..5444ead 100644 --- a/src/game/playerbot/PlayerbotAI.cpp +++ b/src/game/playerbot/PlayerbotAI.cpp @@ -827,6 +827,34 @@ void PlayerbotAI::HandleBotOutgoingPacket(const WorldPacket& packet) return; } + // if someone tries to resurrect, then accept + case SMSG_RESURRECT_REQUEST: + { + if (!m_bot->isAlive() && m_botState != BOTSTATE_DEADRELEASED) + { + WorldPacket p(packet); + uint64 guid; + p >> guid; + uint32 namelen; + p >> namelen; + + std::string name; + p >> name; + p >> Unused<uint8>(); + + uint8 isPlayer; + p >> isPlayer; + + WorldPacket* const packet = new WorldPacket(CMSG_RESURRECT_RESPONSE, 8+1); + *packet << guid; + *packet << uint8(1); // accept + m_bot->GetSession()->QueuePacket(packet); // queue the packet to get around race condition + + // set back to normal + SetState(BOTSTATE_NORMAL); + } + } + /* uncomment this and your bots will tell you all their outgoing packet opcode names case SMSG_MONSTER_MOVE: case SMSG_UPDATE_WORLD_STATE: diff --git a/src/game/playerbot/PlayerbotPriestAI.cpp b/src/game/playerbot/PlayerbotPriestAI.cpp index 38aba57..69938d3 100644 --- a/src/game/playerbot/PlayerbotPriestAI.cpp +++ b/src/game/playerbot/PlayerbotPriestAI.cpp @@ -437,7 +437,7 @@ void PlayerbotPriestAI::DoNonCombatActions() continue; // first rezz em - if (!tPlayer->isAlive() && !tPlayer->GetPlayerbotAI()) + if (!tPlayer->isAlive()) { std::string msg = "Resurrecting "; msg += tPlayer->GetName(); This makes it possible for a bot to accept resurrection and for a bot to resurrect a bot within the party. It tested fine for me, but test it for yourself as well; it is easy to miss something. I suppose it might be nice if the bot was to be thankful...
-
This part fixes the Priest resurrecting: diff --git a/src/game/playerbot/PlayerbotPriestAI.cpp b/src/game/playerbot/PlayerbotPriestAI.cpp index 9ac7c54..38aba57 100644 --- a/src/game/playerbot/PlayerbotPriestAI.cpp +++ b/src/game/playerbot/PlayerbotPriestAI.cpp @@ -388,7 +388,7 @@ void PlayerbotPriestAI::DoNonCombatActions() // buff master if (POWER_WORD_FORTITUDE > 0) - (!GetMaster()->HasAura(POWER_WORD_FORTITUDE, EFFECT_INDEX_0) && ai->CastSpell(POWER_WORD_FORTITUDE, *(GetMaster()))); + (!GetMaster()->HasAura(POWER_WORD_FORTITUDE, EFFECT_INDEX_0) && GetMaster()->isAlive() && ai->CastSpell(POWER_WORD_FORTITUDE, *(GetMaster()))); // mana check if (m_bot->getStandState() != UNIT_STAND_STATE_STAND) Apparently, buffs are removed when dead, so the bot was trying to rebuff which prevented the resurrect spell from being cast.
-
There appears to be a problem with the bots mounting up after the master mounts. The problem code in PlayerBotAI.cpp is: case SMSG_FORCE_RUN_SPEED_CHANGE: { WorldPacket p(packet); - uint64 guid = extractGuid(p); + uint64 guid = p.readPackGUID(); if (guid != GetMaster()->GetGUID()) return; if (GetMaster()->IsMounted() && !m_bot->IsMounted()) There might be some other instances of where a packed GUID is sent in the packet, but is not correctly read in these packets. I think it was working before.
-
Multiple host service
BThallid replied to Auntie Mangos's topic in OldInstallation, configuration & upgrades
There is something wrong there. Is there anything else using the port you have specified for the world server? -
I helped a bit on that. I haven't had the chance to do any recent web dev'ing recently. After you switched to the new .net platform is where I stopped at. I haven't worked on it since nor have I worked on my personal web project (grocery cost tracking). I think I got a little side tracked learning C++ a bit on the server and on pseuwow. It's a bad habit of mine.
-
In src/game/ReputationMgr.cpp, I added a function called SetBaseDefaults that is called before and after the player's faction is changed. Comment out the section: if (faction->Standing != 0) { faction->Standing = 0; faction->Changed = true; } You will need to keep the section below that though to make sure the flags for AtWar and such are set.
-
More specifically from the 'traitor' branch. So it would be 'git pull git://github.com/BThallid/mangos.git traitor' BTW: Branch recently updated to allow riding training while a traitor. I had to do a hack so to speak to work around the client's restriction of not allowing the use of the item that teaches the mount. It will teach the purchased mount upon purchase and destroy the item, unless they already know the mount, then it will not destroy the item. In case someone buys the mount over and over.
-
I just did a merge with 10288 for my branch. It was conflicting back from 1a2b30f2 [10270].
-
WorldSocket::handle_input_header: client sent malformed packet size
BThallid replied to a topic in OldGeneral support
Perhaps it would be helpful if this message contained the IP address. - sLog.outError ("WorldSocket::handle_input_header: client sent malformed packet size = %d , cmd = %d", - header.size, header.cmd); + sLog.outError ("WorldSocket::handle_input_header: client '%s' sent malformed packet size = %d , cmd = %d", + GetRemoteAddress().c_str(), header.size, header.cmd); ^Not tested, but should do it. I think some website function may cause this when the website is accessed and it checks if the server is online. -
Client's AuthSession did change in http://github.com/mangos/mangos/commit/ae728907a531d918030a066c86248547277ff58a
-
[Help/Bug] High load performance bug. Could be camera system problem.
BThallid replied to a topic in OldBug reports
If that's bandwidth spikes, I would be more concerned about how there's less activity just before a spike. The spike would be the server just sending all the packets that get queued while it was busy. Is 31.488 Avg Self Time normal for 186 Visits for Player::LoadFromDB? Is the DB running in 64-bit or 32-bit? -
That right there is why I think a lot of devs just get up a leave a project. If someone wants something, learn to do it yourself or just be patient. I wouldn't blame Vlad, Tom, or any of the devs for stopping--why spend their free time on a project if they just hear complaints. I would have to admit, however, that it would be nice to know what kind of roadmap there is of what they are wanting fixed in the existing code. I've seen some mention of a few things, but can't remember them completely. Sorry for the off topic posts Lynx, but it seemed the perfect opportunity.
-
I am too humble to accept any praise. I have posted it to show that anyone willing to try can learn. I am only a beginner with C++; if there are better ways to do anything that I have done, please improve upon it. The client is still restricting quite a bit, hence the bugs that may rear their ugly heads.
-
The original design was to make it so that when Alliance players joined a guild led by a Horde player it would then make them switch when they joined.
-
What's it do? This patch allows for players to become a traitor to their original race's team. Imagine running around as a Tauren for the Alliance or a Gnome in the Horde. It is intended as a fun modification. The healing, quests, vendors, and most things work for the traitor players. How to use it? The patch requires AllowTwoSide.Interaction.Guild = 1 in the config to allow a player to change without GM intervention by joining the enemy guild. You may want to enable a couple of the other AllowTwoSide options such as chat. The GM command is .character switchteam for manual switching. The player will not be teleported to a safe zone, so once they are switched, they will be attacked if there are guards. There is an additional column added to the characters table in the db to store the faction code of the race. If it doesn't match their original faction or zero, they are considered a traitor. I originally intended it to be used to switch between any of the factions. Be sure to add it from the sql/updates/patchtraitor_characters_characters.sql. What's wrong with it? Apart from the fact that the Horde races might be running around in the Alliance, there are a couple of bugs. Ones that are mostly clientside to the best of my knowledge. I don't believe there is a work around for them, but have not thoroughly checked myself. * Mages cannot get portal training for enemy team (data is sent) * Some NPC text appears in different language even though you might know the language (a flag for the NPC?) * Client will not show other languages as a choice to chat with (even with the language skill) * While in PvP mode, the original team is display on your own portrait instead of the team you are a member of (shows correctly for others) * PvP achievements may not work correctly (haven't tested) * Mount vendor NPC gossip for scriptdev requires a work around inside of /scripts/world/npcs_special.cpp to allow mounts to be purchased Other Caveats Upon switching sides, it will clear out the current reputation. Technically, this doesn't have to be done, but I thought it a good punishment for becoming a traitor. Remember, I am still learning. If anyone has ways to improve upon this, have at it! Have fun! Where to get it? For current core: With git or browse: git pull git://github.com/BThallid/mangos.git traitor For Zero: With git or browse: git pull git://github.com/BThallid/mangos-zero.git traitor-zero For One: With git or browse: git pull git://github.com/BThallid/mangos-one.git traitor
-
Apparently, when copy and pasting the code to a file using something like notepad will give whitespace errors which can be ignored or rather fixed with the option --whitespace=fix when using git apply. The fatal part is caused by the need for a new line ending. Just enter/return down one line at the end.
-
I have no conflicts with applying my patch to fresh rev 9763. Are you talking about the branch that Thyros mentioned in insider42 repo? Do you have other patches? More details help problem solving.
-
He has the chatlog/lexicscutter version. Mine is only a chatlog. I meant for it to be simple, but I think code starts to grow over time. Updated with rev 9748. Don't know what changed; I just rebased it and it didn't give me any errors. I compiled it with rev 9745 with no problems a little while back. I don't see any changes in the most recent commits that would effect it, so it should still compile just fine.
-
I'm not sure of what you mean. I thought I was using almost the same as what I was seeing used for the way the gmlog was made. If there is a better way, by all means have at it. I was thinking about using the shared defines header, but as I am still learning about the various part of C, I didn't want to add the header for just one thing if it would be degrading more. I had finished the update a few days ago and intended on researching it further to find out the best method, but I had got busy with that whole real life stuff that's around.
-
Sorry, beat you to it. First post modified with requested features.
Contact Us
To contact us
click here
You can also email us at [email protected]
Privacy Policy | Terms & Conditions

You can also email us at [email protected]
Privacy Policy | Terms & Conditions
Copyright © getMaNGOS. All rights Reserved.
This website is in no way associated with or endorsed by Blizzard Entertainment®
This website is in no way associated with or endorsed by Blizzard Entertainment®