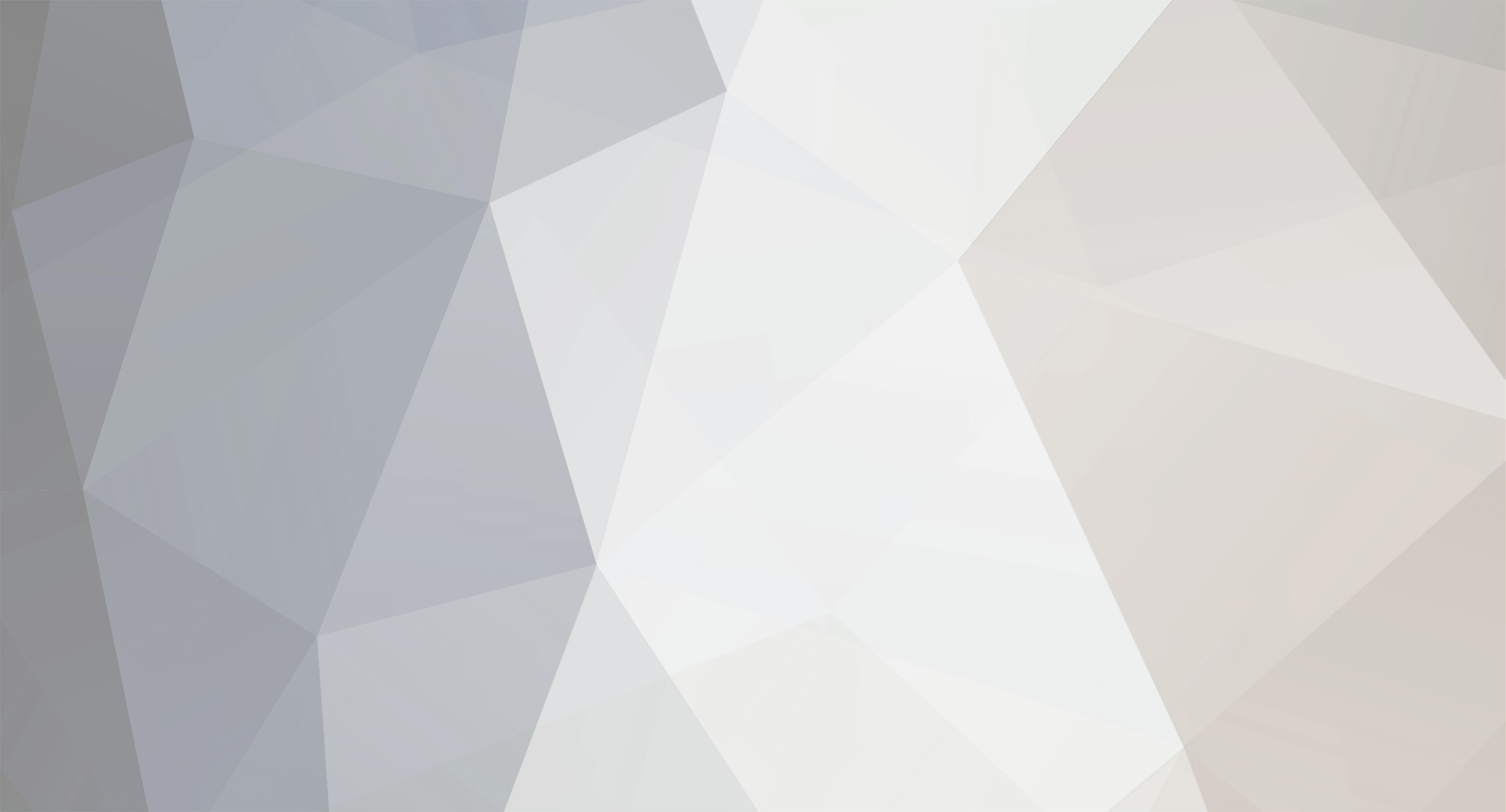
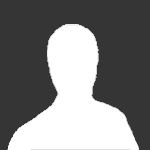
darkstalker
-
Posts
717 -
Joined
-
Last visited
Never -
Donations
0.00 GBP
Content Type
Profiles
Bug Tracker
Wiki
Release Notes
Forums
Downloads
Blogs
Events
Posts posted by darkstalker
-
-
eye is not a vehicle, its a charmed unit
-
what does this exactly do?
-
probably the flight path "continues while offline", since getting instantly to destination after forced logout looks exploitable
-
setting "GridUnload = 0" increases stability, but breaks mob respawn times.
-
TBB is used on all the C++ memory allocation (new, delete) since if redefines the global "void* operator new" (see framework/Policies/MemoryManagement.cpp). This overrides default standard library memory allocation with a custom one. Its a link-time process.
Two kinds of memory management can coexist if you don't mix them (like allocating with malloc and freeing with operator delete). Probably the detour library comes with an "optimized malloc" for people who doesn't care about memory management, but in our case we doing it, so the best would be disable it so it uses TBB. Maybe this will require extra work since if you're using malloc-like functions to get memory then you need placement new to create objects on it.
-
i think someone was working on this already, don't know if he had success or not
-
easier to work with 2 numbers than 3, would make templates more complex. Besides using 64bit values saves a bit of cpu cycles on 64 bit machines (anyone still uses 32 bits?)
-
This is an in-progress implementation of a bitset-like class that wraps the 96 bit SpellFamilyFlags + SpellFamilyFlags2 into a single object that can be accessed by individual bit position. Its implemented via template metaprogramming in order to achieve compile-time bit mask calculation in a transparent way with no overhead. This gives a more readable and verbose version of stuff like:
case SPELLFAMILY_WARLOCK: // For Hellfire Effect / Rain of Fire / Seed of Corruption triggers need do it if (m_spellInfo->SpellFamilyFlags & UI64LIT(0x0000800000000060)) m_canTrigger = true; break; case SPELLFAMILY_PRIEST: // For Penance,Mind Sear,Mind Flay heal/damage triggers need do it if (m_spellInfo->SpellFamilyFlags & UI64LIT(0x0001800000800000) || (m_spellInfo->SpellFamilyFlags2 & 0x00000040)) m_canTrigger = true; break;
that becomes:
case SPELLFAMILY_WARLOCK: // For Hellfire Effect / Rain of Fire / Seed of Corruption triggers need do it if (m_spellInfo->SpellClassMask.test<CF_WARLOCK_RAIN_OF_FIRE, CF_WARLOCK_HELLFIRE, CF_WARLOCK_SEED_OF_CORRUPTION2>()) m_canTrigger = true; break; case SPELLFAMILY_PRIEST: // For Penance,Mind Sear,Mind Flay heal/damage triggers need do it if (m_spellInfo->SpellClassMask.test<CF_PRIEST_MIND_FLAY1, CF_PRIEST_PENANCE_DMG, CF_PRIEST_PENANCE_HEAL, CF_PRIEST_MIND_FLAY2>()) m_canTrigger = true; break;
Where CF_* is an enum that associates the bit position with a name like:
enum CLASS_FLAG { CF_MAGE_FIREBALL = 0, CF_MAGE_FIRE_BLAST = 1, CF_MAGE_FLAMESTRIKE = 2, CF_MAGE_FIRE_WARD = 3, CF_MAGE_SCORCH = 4, CF_MAGE_FROSTBOLT = 5, // ...
Source code with the class here: http://paste2.org/p/1094906
-
doing a SQL query each time you cast a spell is cumbersome, you should read data from in memory structure, or better use DBC to get bounding box of the gameobject
-
./configure --help
-
target == (TargetInfo*)0x10
doing this makes no sense
-
maybe it should have a default icd
-
there is a config option to change visibility distance
-
its related to some movement flag i think
-
its currently vulnerable to stun, invulnerable to disarm (should be both the opposite)
-
there are some guides around based on the "item save fail" method
-
don't know if still applicable.
currently i saw some crashes in EventProcessor related to grid unload
-
you're force-applying each update right?
would be interesting to make an updater that asks mangos version via SOAP and then calculates exactly what is needed
-
you can add an extra function after DBC loading that does changes in-memory
-
procs from sap too..
-
i got my personal dbc browser, but still on early development
-
for scroll case yes, but there are tons of auras that should stack visually, but the net effect should be only the maximum value of the category, example: resistance buffs
-
i could but don't know how to..
something is missing there, like a formula to correlate DBC data to expected values, now i just see magic numbers
-
looks like a C implemetation of what would be std::deque<bool> in C++
It isn't. It works more like C++ streams than any container.
double ended queues are used to implement streams
[BUG] Problems with target-type (22, 7)
in OldBug reports
Posted
i think there was a positive-negative problem here